Starter project overview - project structure
Note that the folder structure in this lesson is the same as in the starter project, but it does not match the one on the latest GitHub repo. This is because we will change the folder structure later in section 3 about app architecture.
To avoid confusion, follow the starter project for now.
main.dart
This is where we run the app and load the root widget of the entire app:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
// * Register error handlers. For more info, see:
// * https://docs.flutter.dev/testing/errors
registerErrorHandlers();
// * Entry point of the app
runApp(const MyApp());
}
void registerErrorHandlers() {
// * Show some error UI if any uncaught exception happens
FlutterError.onError = (FlutterErrorDetails details) {
FlutterError.presentError(details);
debugPrint(details.toString());
};
// * Handle errors from the underlying platform/OS
PlatformDispatcher.instance.onError = (Object error, StackTrace stack) {
debugPrint(error.toString());
return true;
};
// * Show some error UI when any widget in the app fails to build
ErrorWidget.builder = (FlutterErrorDetails details) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.red,
title: Text('An error occurred'.hardcoded),
),
body: Center(child: Text(details.toString())),
);
};
}
For more info about the error handling code, read:
MyApp widget
This is the root widget of the entire app:
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
restorationScopeId: 'app',
// * The home page of the app
home: const ProductsListScreen(),
onGenerateTitle: (BuildContext context) => 'My Shop'.hardcoded,
theme: ThemeData(
// * Use this to toggle Material 3 (defaults to true since Flutter 3.16)
useMaterial3: true,
primarySwatch: Colors.grey,
appBarTheme: const AppBarTheme(
backgroundColor: Colors.black87,
foregroundColor: Colors.white,
elevation: 0,
),
elevatedButtonTheme: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.black, // background (button) color
foregroundColor: Colors.white, // foreground (text) color
),
),
),
);
}
}
Note about Material 3
Since Flutter 3.16, useMaterial3
is set to true
by default.
So keep in mind that when you run the app, you’ll see the latest Material 3 UI:
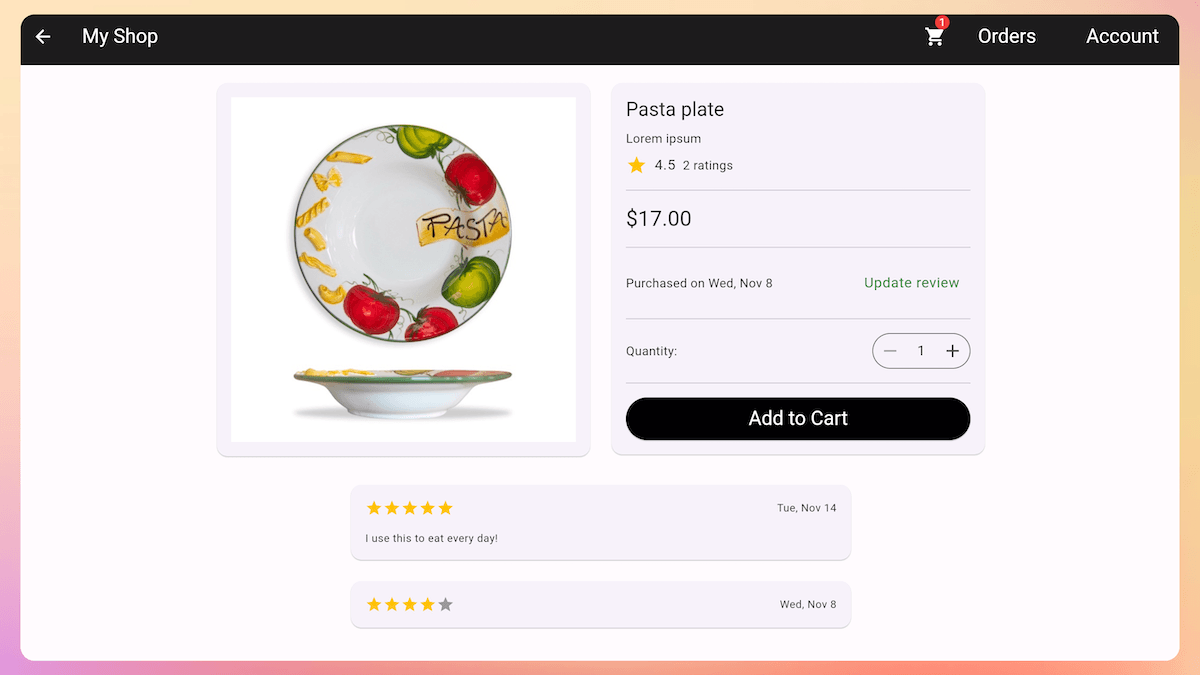
But most of the course lessons were recorded before Flutter 3.16 and still show the old UI.
Project folders
Here is how the top-level folders are organized:
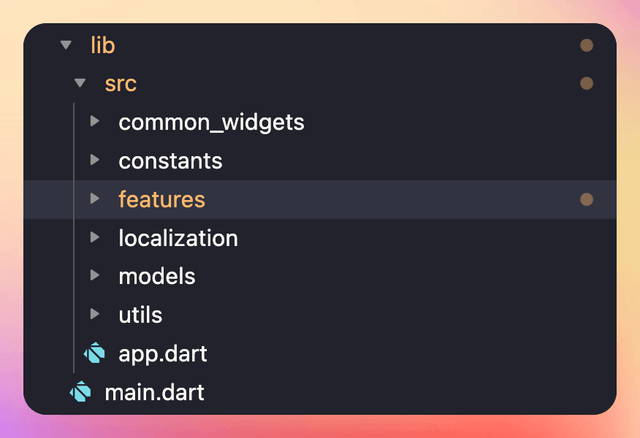
The most important folders discussed in the video are features
and models
.
Upcoming project structure changes
The features
folder inside the starter project looks like this:
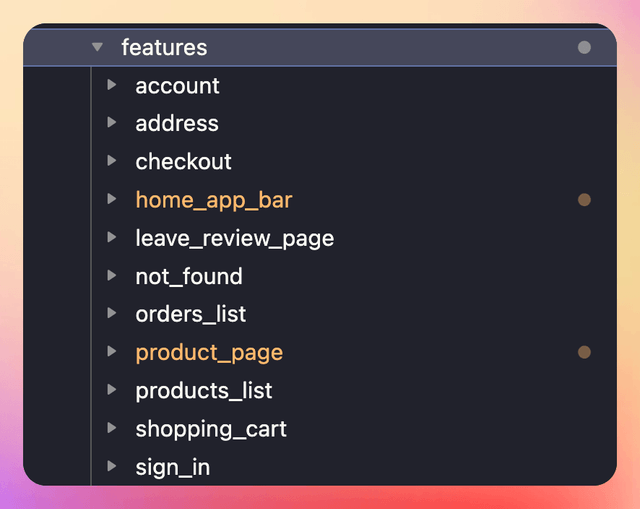
However, the completed project on GitHub does not match this. That’s because we will adopt a different project structure from module 3 onwards.
Project Constants
Many of the constants in the project are defined in the app_sizes.dart
file:
import 'package:flutter/material.dart';
/// Constant sizes to be used in the app (paddings, gaps, rounded corners etc.)
class Sizes {
static const p4 = 4.0;
static const p8 = 8.0;
static const p12 = 12.0;
static const p16 = 16.0;
static const p20 = 20.0;
static const p24 = 24.0;
static const p32 = 32.0;
static const p48 = 48.0;
static const p64 = 64.0;
}
/// Constant gap widths
const gapW4 = SizedBox(width: Sizes.p4);
const gapW8 = SizedBox(width: Sizes.p8);
const gapW12 = SizedBox(width: Sizes.p12);
const gapW16 = SizedBox(width: Sizes.p16);
const gapW20 = SizedBox(width: Sizes.p20);
const gapW24 = SizedBox(width: Sizes.p24);
const gapW32 = SizedBox(width: Sizes.p32);
const gapW48 = SizedBox(width: Sizes.p48);
const gapW64 = SizedBox(width: Sizes.p64);
/// Constant gap heights
const gapH4 = SizedBox(height: Sizes.p4);
const gapH8 = SizedBox(height: Sizes.p8);
const gapH12 = SizedBox(height: Sizes.p12);
const gapH16 = SizedBox(height: Sizes.p16);
const gapH20 = SizedBox(height: Sizes.p20);
const gapH24 = SizedBox(height: Sizes.p24);
const gapH32 = SizedBox(height: Sizes.p32);
const gapH48 = SizedBox(height: Sizes.p48);
const gapH64 = SizedBox(height: Sizes.p64);