State Management Basics
With over 40 packages available, state management is likely the hottest topic in Flutter.
Don’t believe me? Here’s the full list on Flutter Gems:
Fear not, since today I’ll tell you what you need to know about state management - without all the noise.
State Management
Flutter is a declarative framework in that it builds the UI to reflect the current state of your app:
UI = f(state)
This is briefly explained in this page called Start thinking declaratively.
But what is state exactly? The Flutter documentation defines state as:
whatever data you need in order to rebuild your UI at any moment in time
It also introduces a clear distinction between app state and ephemeral (or local) state:
As a rule of thumb, you can use setState
and the StatefulWidget
class when dealing with local widget state. This keeps things simple.
But when it comes to state that needs to be shared across multiple widgets, there are multiple approaches and techniques that you can use.
So, what’s the best way to get started?
Flutter Widgets 101
This series covers the basics of state management in Flutter. Make sure you watch and understand this before moving to any of the more complex solutions:
- How to Create Stateless Widgets - Flutter Widgets 101 Ep. 1
- How Stateful Widgets Are Used Best - Flutter Widgets 101 Ep. 2
- Inherited Widgets Explained - Flutter Widgets 101 Ep. 3
- When to Use Keys - Flutter Widgets 101 Ep. 4
You can go pretty far and build non-trivial applications with the built-in StatelessWidget
, StatefulWidget
, and InheritedWidget
classes. More importantly, it’s critical to understand the foundations of state management before you can move to more complex solutions.
For a comprehensive overview of Flutter’s built-in tools for state management, you can also check this:
Daily Challenge - Multiple Counters
To get some practice with Flutter’s built-in tools for state management, I prepared the following challenge.
Extend the default Flutter counter app to work with multiple counters so that it looks like this:
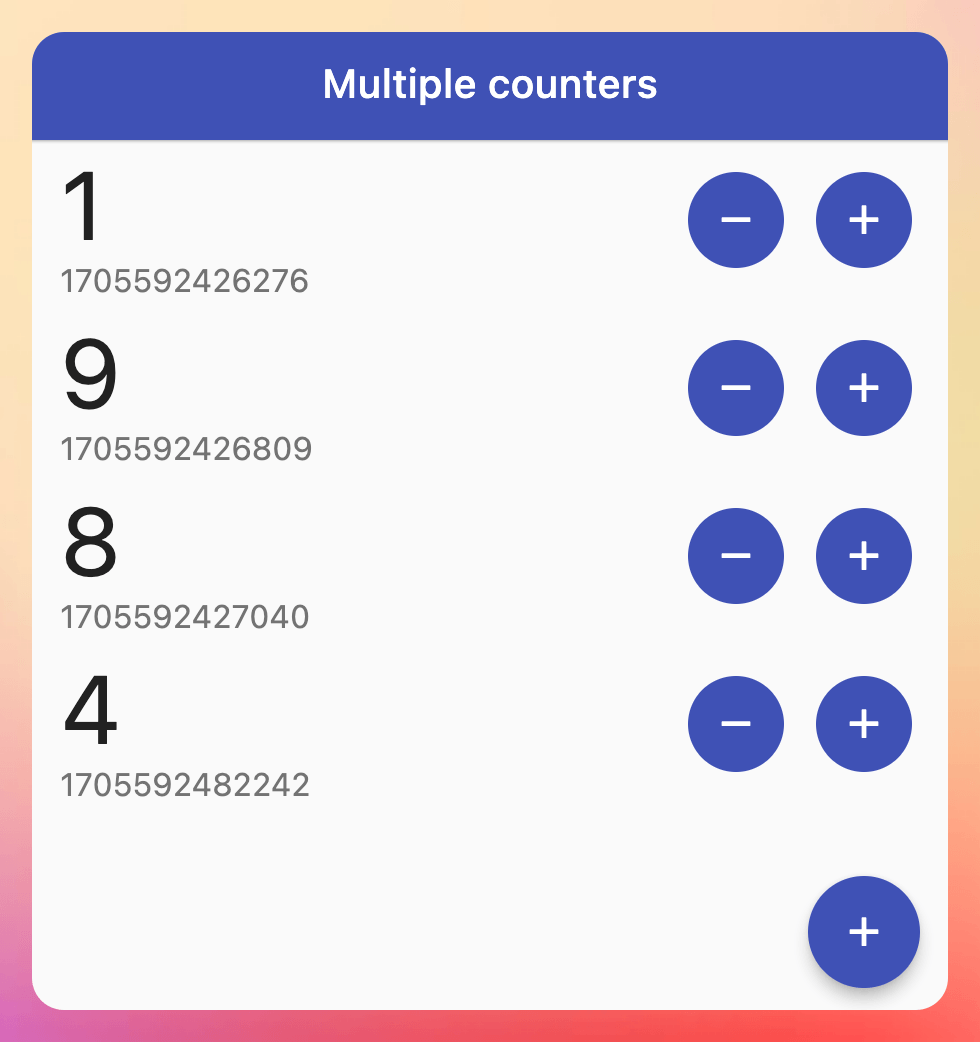
The resulting app should have:
- a floating action button that you can use to add a new counter
- a
ListView
that shows all the counters - each item in the
ListView
should show the counter value and have buttons to increment/decrement the counter value
For this challenge, you can store all the counter values as local application state (no need to persist them to local storage).
Daily Challenge - TODO App
If you’re up for a bigger challenge, try building a TODO app such as this:
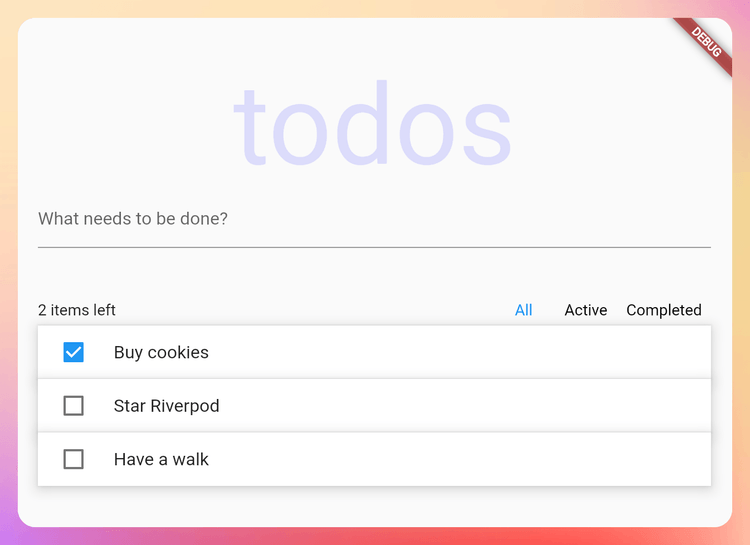
In the next lesson, we’ll learn about more complex and powerful state management solutions.
Happy coding!