State Management Packages & App Architecture
Once you’re feeling confident with the built-in state management APIs in Flutter, you can start looking at some 3rd party solutions.
Here, I focus on the most stable, well-documented, maintained, and supported packages. The ones I used personally and can recommend without hesitation are:
Other honourable mentions are signals.dart, rxdart, get_it, stacked, mobx, states_rebuilder and redux.
But you know what? You only need one of these, and only after you’ve covered the basics from the previous lesson.
Provider
Simply put, Provider = InheritedWidget + Generics. Provider gives you scoped access to things in your widget tree by type. The Flutter team officially endorses it. It’s very mature, and you’ll find a lot of documentation and Q&A on StackOverflow about it.
I recommend my Flutter Provider video series on YouTube for a good conceptual overview of Provider.
flutter_bloc
Just like Provider, flutter_bloc is a very mature and robust solution for state management and has been around for many years.
If you head over to bloclibrary.dev, you’ll find some very good documentation covering all the most important core concepts, along with tutorials showing how to build real apps with full source code.
The earlier versions of flutter_bloc
were criticized for requiring too much boilerplate code. But since then, the package also includes cubit
, a lightweight version of bloc.
The best place to learn Flutter Bloc (along with many important concepts) is this 3-hour free course on YouTube:
Riverpod
Riverpod is a reactive caching and data-binding framework that was born as an evolution of the Provider package.
It borrows many concepts from Provider but is fundamentally different in that it does not depend on Flutter and the widget tree. Riverpod uses global providers that are referenced by name, not type. This is a big departure from a conceptual point of view.
Riverpod allows you to manage state in complex applications in a type-safe way with minimal boilerplate code. It has great support for testing and works really well when combining providers together.
Head to riverpod.dev to read the official documentation and browse the example apps.
My ultimate guide to Riverpod covers everything you need to know:
And if you want to go deeper, you can check out all the remaining Riverpod articles on my site:
Which Package is Best?
Ultimately, all these packages help you separate your business logic from the UI code.
For a direct comparison of Provider, flutter_bloc, and Riverpod, you can check this movie app that I’ve built using all three packages:
Having used Provider, flutter_bloc and Riverpod in my projects, I can say that Riverpod is by far my favorite. If your app needs data caching and reactive updates, you can’t go wrong with it.
All these packages are great, but don’t let my perspective influence your decision. Many other packages are also very good. If you come from JavaScript and you’re familiar with redux, then that may be the right solution for you. After all, tools are only valuable if you know how to use them correctly.
What about App Architecture?
The state management solutions above are tools that you can use to build your apps.
But when building complex apps, choosing the correct app architecture is crucial, as it allows you to structure your code and support your codebase as it grows.
In building Flutter apps over the last few years, I’ve learned many lessons. And I ended up creating my own app architecture, which is now used by many other Flutter devs.
You can learn all about it here:
But there’s more. If we want to create complex apps with many pages and features, we should decide how to structure our project.
This ensures that the entire team can follow a clear convention and add features in a consistent manner.
So here’s an overview of two of the most common approaches:
Summary
Without a doubt, state management & app architecture are broad topics, and it can be challenging to “get it right” when building complex apps.
So, if you’re just getting started, my advice is to see how far you can get with Flutter’s built-in state management solutions.
Once you have a good understanding of the basics, consider giving Riverpod a try.
And if you want to go deeper, I recommend taking my Flutter Foundations course, where you’ll learn about state management, app architecture, navigation, testing, and much more by building a full-stack Flutter eCommerce app on iOS, Android, and web:
Daily Challenge - Refactor the counters app
Yesterday’s challenge was to extend the standard Flutter counter app to work with multiple counters.
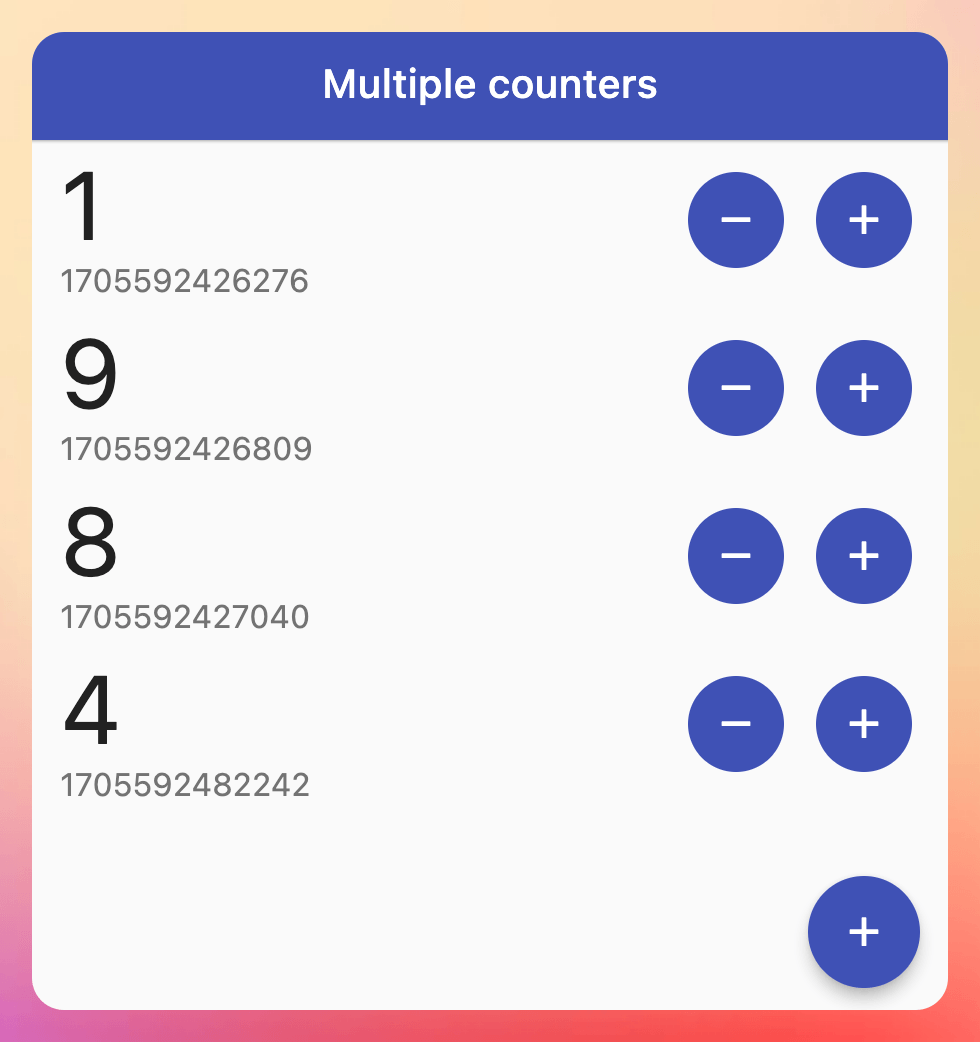
Here’s the brief once again:
- add a floating action button that you can use to add a new counter
- add a
ListView
that shows all the counters - each item in the
ListView
should show the counter value and have buttons to increment/decrement the counter value
Today’s challenge is to refactor this app to use your favourite state management package.
Both flutter_bloc and Riverpod already include sample code showing how to build a single counter. You can use this as a basis for this project:
Good luck, and see you in the next lesson!