Interactivity & User Input
Every app needs to deal with user input in one way or another.
For example, consider this email & password sign-in form:
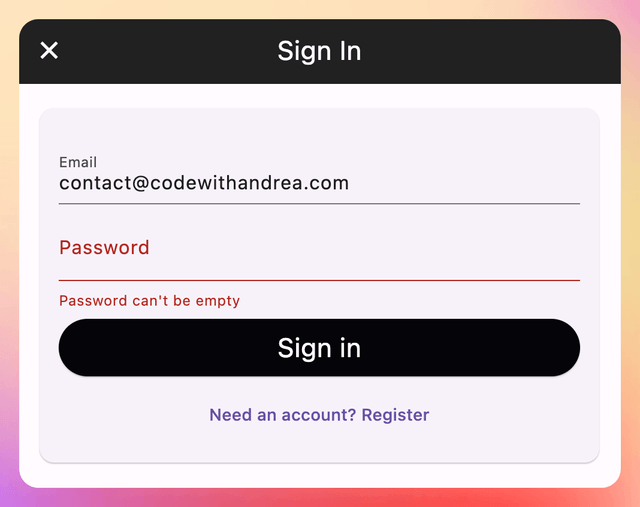
Building this should be simple, right?
As it turns out, there are many details to consider:
- input text validation
- handling the selected focus and dismissing the keyboard when needed
- getting and setting the text fields’ data and updating the form state
- text field appearance (decoration, placeholder text, error hints etc.)
You can build the email and password fields above with some TextField
widgets.
Because Flutter is declarative, you need to pass a TextEditingController
as an argument to TextField
and use it to control the text inside it. This is typically done inside a StatefulWidget
subclass that is the parent of your TextField
(s).
However, if you have more than one text field on your page, you’ll need a TextEditingController
for each TextField
, and this can lead to a lot of boilerplate.
One way to make your life easier is to use the built-in Form
and FormTextField
widgets, and this tutorial explains how to do so:
Note:
FormTextField
usesTextField
under the hood.TextField
is a very customizable widget with many useful properties.
The best place to learn how to use all these input widgets is the Flutter forms cookbook, which includes various interactive examples:
TextField
is quite powerful, and you can learn all about it here:
And if you want to learn how to do client-side validation, I’ve got you covered with this article:
Too Much Boilerplate?
Working with TextEditingController
can add a lot of boilerplate to your apps. [flutter_hooks](https://pub.dev/packages/flutter_hooks)
provides an elegant solution for this and comes with a useTextEditingController API that you can use to streamline your text editing code.
You can see an example of how to do this in my BMI calculation app on GitHub.
More Than Just Text Fields
Text input is just one of many ways users can interact with our app.
Other common input widgets are date and time pickers, checkboxes, radio boxes, sliders, and switches.
These are all listed as part of the Material Components widgets catalog.
And if you’re targeting iOS, you can also use the corresponding Cupertino (iOS-style) widgets.
Gestures
All the widgets we discussed above are visual UI components that you can show on screen. But Flutter also gives you some rich APIs for detecting user gestures.
For a good overview of how to listen for and respond to gestures in Flutter, see this page:
And if you want to take a deep dive, this article has you covered:
Daily Challenge - BMI Calculator
Build a BMI calculator app that lets the user input his/her weight and height and shows the corresponding BMI.
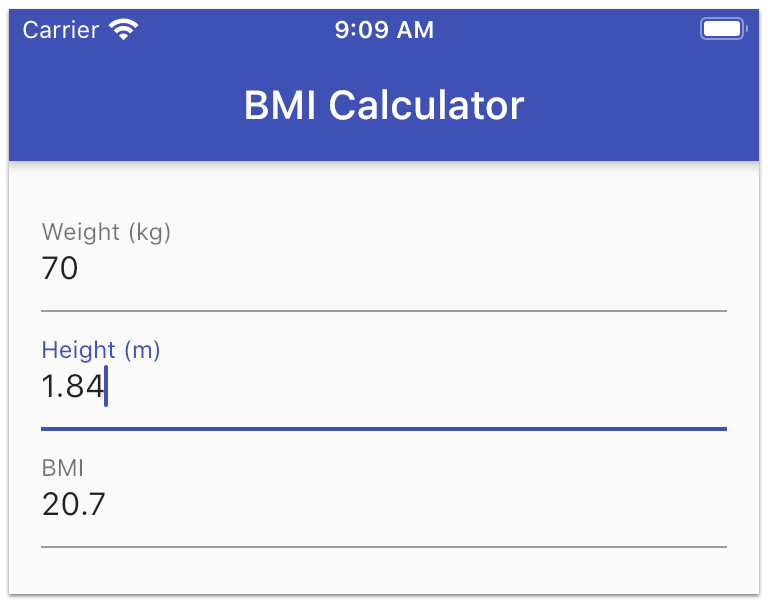
If you need some guidance and sample code to work with, you can check out this tutorial, which uses a BMI calculation app as an example:
Happy coding!