Intro to Error Monitoring
In this module, you’ll learn how to monitor runtime errors in your Flutter apps using tools like Sentry and Crashlytics.
We’ll cover everything from setup to debugging real production issues—so you can ship confidently and respond quickly when things go wrong.
But first, let’s ask a simple question:
Why Do You Need Error Monitoring?
If your app throws an exception during development, the debugger will scream at you:
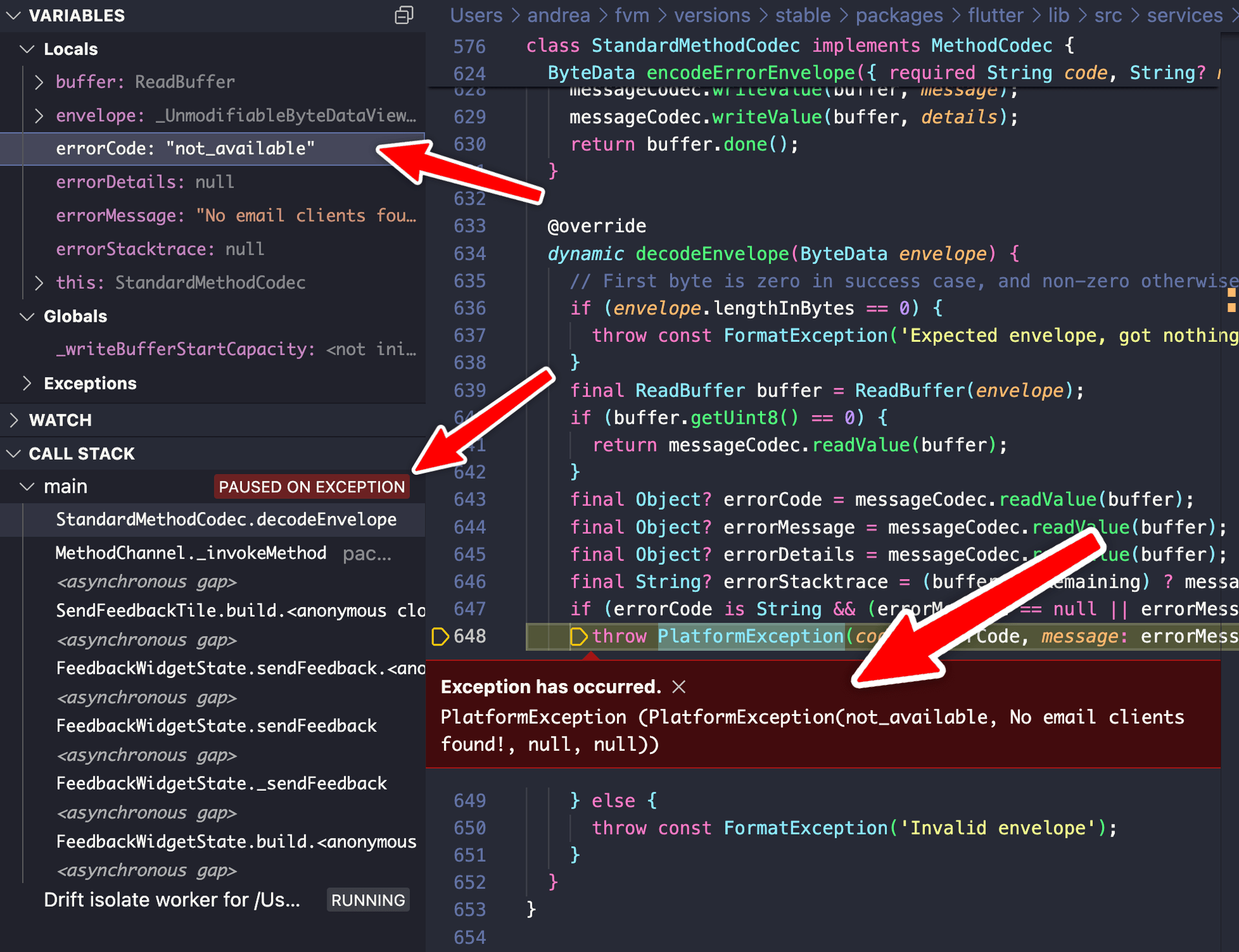
In this scenario:
- You can see the exact file and line where the exception was thrown
- You have the full stack trace
- You can inspect variables and step through the code
- You understand the sequence of events leading up to the exception
This is the best possible debugging environment.
But What Happens in Production?
Once your app is in the hands of real users, you lose that visibility.
If the app crashes, you don’t get logs. You don’t get exceptions. You don’t even know it happened—unless your users tell you (99% of them won’t).
Without monitoring, you’re flying blind.
You won’t know:
- What issues are most frequent
- What is their impact
- Which app versions are affected
- On which devices and OS versions they occur
- What the user was doing before the crash
This is where tools like Sentry and Crashlytics come in—they capture this data for you.
When should you add error monitoring?
Add it before your first production release so you can monitor issues from day one.
If your app is already live, do yourself a favor and add it in your next release. Seriously.
Over time, you’ll reduce the number of crashes and improve stability. With a proactive approach, I’ve seen production crash rates drop to nearly zero. ✅
Bonus: during the app review process, your app will be tested on various devices and may be rejected if it crashes. In this scenario, error monitoring can provide invaluable information about what went wrong and how to fix it.
What We’ll Cover
Here’s what you’ll learn in this module:
- 🆚 Sentry vs Crashlytics: When to use each
- 🚀 Setup: How to integrate Sentry and Crashlytics (including environments and flavors)
- 🧠 Sentry Dashboard: Overview and issue resolution workflow
- 📣 User Feedback: How to collect feedback directly from your app
- 🧰 Additional APIs and Options: Logging errors manually, adding navigation and HTTP breadcrumbs, and more
- 🧵 Error Types: How to handle uncaught exceptions with
FlutterError
andPlatformDispatcher
- 🏗️ Build Modes: Why stack traces become unreadable in release builds
- 🔍 Debug Symbols & Source Maps: What they are and how to upload them
By the end, you’ll have a complete error monitoring setup—and a solid plan for tracking and fixing real-world issues.
You’ll also get a setup checklist you can reuse across projects.
Time for a quick quiz!
Question 1 of 3You scored % ( out of 3)
Once you’re ready, let’s get started in the next lesson!