Intro to Force Update
Picture this: you discovered a critical bug that corrupts some data in your app, and you need to fix it ASAP.
If your app is web-based, you can simply deploy the fix, and users will automatically get the latest version as soon as they open it (or refresh their browser tab).
But on mobile, things are different. While iOS and Android offer an “Automatic App Updates” feature, updates are not immediate, and not all users have it enabled.
To ensure 100% of your users are on the latest version, you have two options:
- Implement a Force Update strategy
- Add Code Push to your app to deliver the fix over the air (OTA)
This module will focus on force update strategies you can implement for free in your apps. We’ll cover Flutter code push using Shorebird (a paid tool) in this module.
By adding a force update feature to your app, you can prompt users to update if they are on an old version:
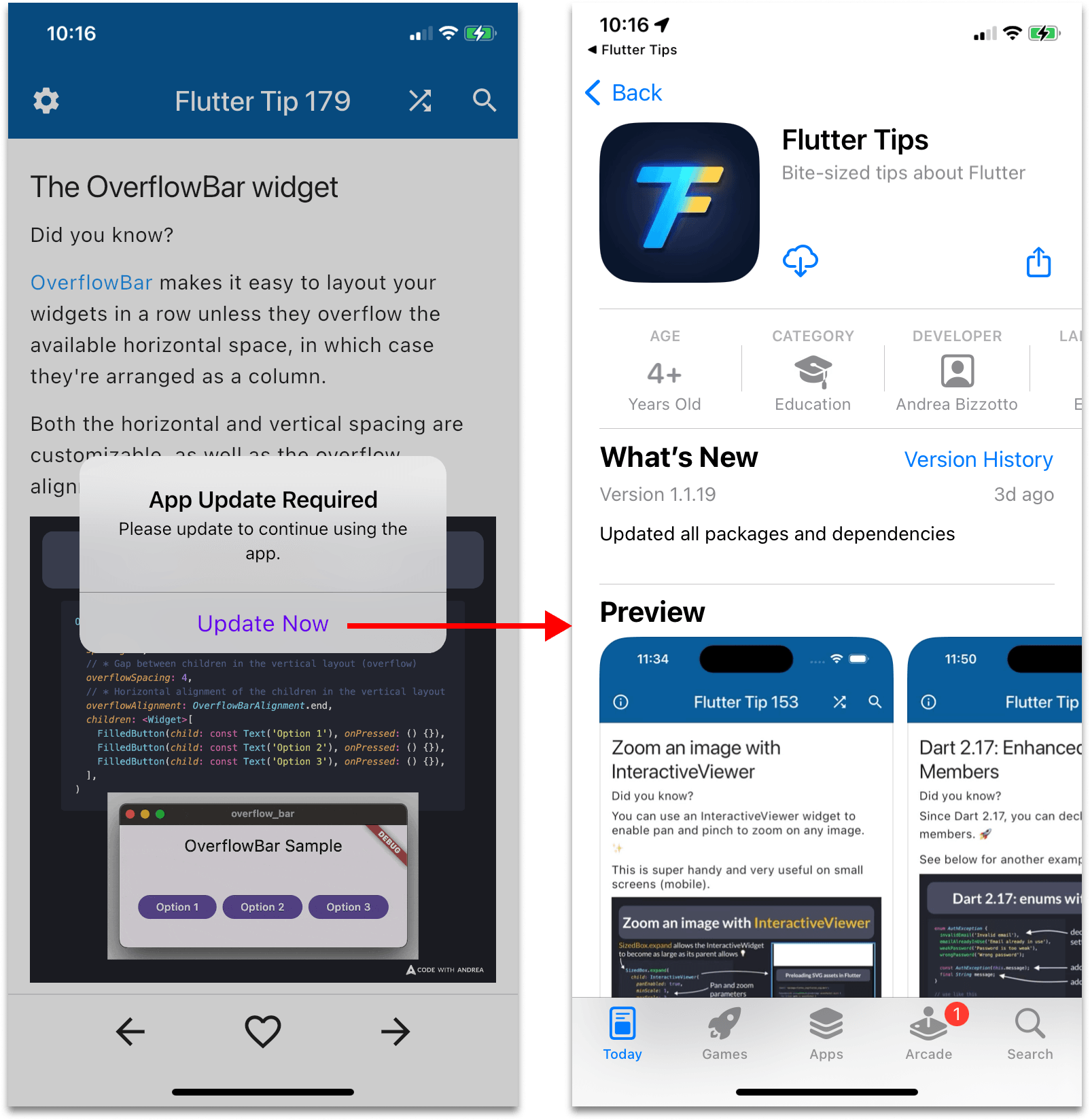
But there’s a catch: force update needs to be implemented in the very first version of your app; otherwise, it won’t work for users on older versions. This diagram illustrates why:
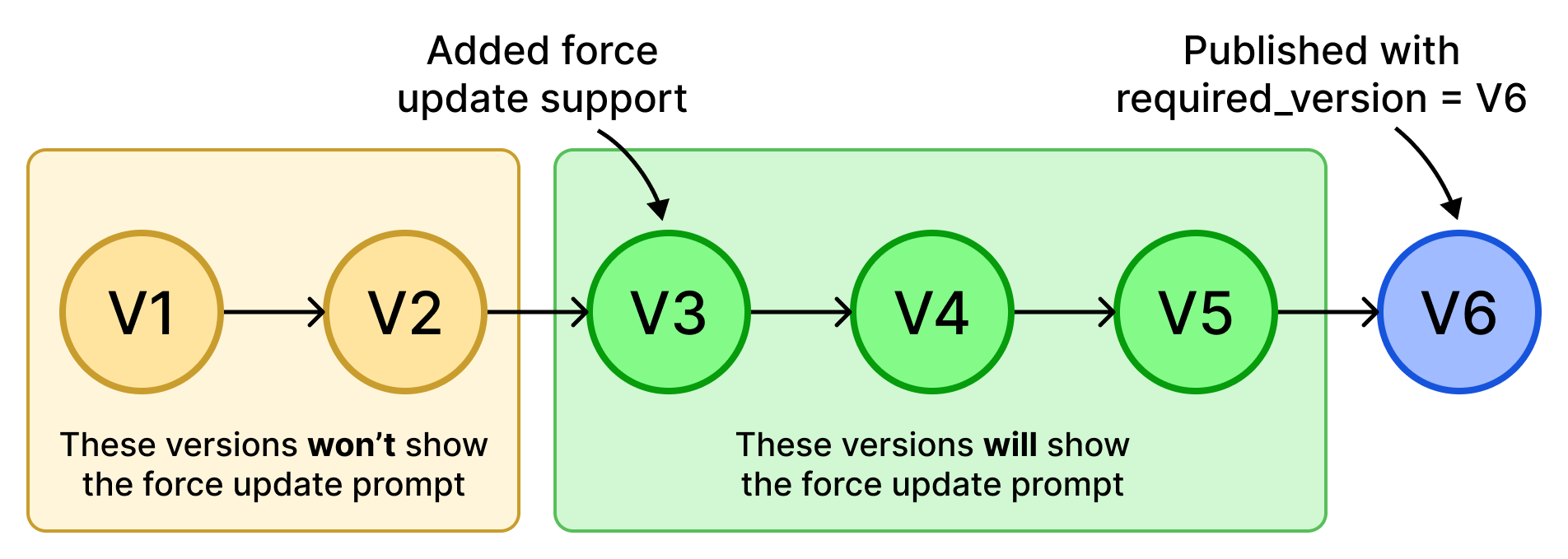
But why do we need force updates in the first place?
The Maintenance Cost of Old App Versions
Without a force update strategy, you can’t assume users will ever be on the latest version of your app.
This has significant implications:
- Users may miss important security updates.
- You can’t retire old backend endpoints because old app versions still use them.
- If you change your backend entirely, you can’t easily migrate all users to the new system.
By enforcing a strict force update policy, you can limit maintenance and testing efforts to app versions within the supported timeframe. This is a big win for your mobile and backend teams. âś…
Overview of Different Force Update Strategies
At a basic level, force update can be implemented by:
- Checking the app’s current version against the minimum required version
- Blocking access to the app if the version is outdated
- Prompting users to update from the app store
This technique can be adjusted to meet different needs:
- Always update when there’s a new version: This approach is used by the upgrader package.
- Update if current version < required version: This allows you to set a minimum required app version without forcing users to always be on the latest.
- Rolling release window: This allows you to define a set of the latest supported app versions, forcing users to update if their current version is not within the allowed range.
- Soft vs hard update: By showing a “soft update alert”, you can inform users that the current version will be deprecated, and they can update now or later.
Force Update: Implementation Options
How can you implement force update in practice? Here are a few options:
- Use the upgrader package, which prompts users to upgrade when there is a newer app version in the store.
- Use the force_update_helper package, which lets you fetch the required version, compare it with the current version, and show the update prompt if needed. You can store the required version remotely in three ways:
- With a GitHub Gist, which returns some JSON with the desired configuration (easiest, but rate limits apply)
- With Firebase Remote Config, by setting a
required_version
flag (ideal if your app already uses Firebase) - With a custom backend that defines a
required_version
endpoint (ideal for apps with a custom backend)
This module will explore all these options, so you can weigh their tradeoffs and choose the most appropriate one for your apps.
Summary
Let’s review the main points of this module with a quick quiz!